This Java source code is an example of a Graphical User Interface (GUI) with different Java Swing GUI elements such as TextBox, Buttons, TextArea, Text Area and Labels. It is implemented using Java Swing API which provides a set of “lightweight” (all-Java language) components. This code example extends the functionality of JFrame class to show a JFrame window and Implements ActionListener on buttons to catch the click event on those buttons.
This Java Swing example uses several Swing components to create a graphical user interface (GUI). Here are the main components used in the code:
- JFrame: The main window or frame of the GUI.
- JComboBox: A dropdown list or combo box for selecting options.
- JLabel: A non-editable text component used to display information or instructions.
- JButton: A button component that triggers actions when clicked.
- JTextArea: A multi-line text area where you can display or input text.
- JScrollPane: A scrollable container that wraps around another component (in this case, the JTextArea) to provide scrolling functionality.
- Container: A generic component that can hold and organize other components.
- JCheckBox: A checkbox that allows the user to toggle a binary choice, such as enabling or disabling a feature. In this example, it’s labeled “Enable Feature.”
- JRadioButton and ButtonGroup:
JRadioButton
components represent radio buttons that are grouped together using aButtonGroup
. Radio buttons within the same group ensure that only one of them can be selected at a time. - FlowLayout: A layout manager that arranges components in a left-to-right, top-to-bottom flow.
- JMenuBar, JMenu, and JMenuItem:
JMenuBar
represents the menu bar at the top of the frame.JMenu
represents a dropdown menu within the menu bar, andJMenuItem
represents individual items within the menu.
These components are used to create a simple interactive program with buttons, a combo box, and text areas, providing a basic understanding of building a GUI in Java.
Java Swing GUI Example Code
Here is the java example code to show Swing GUI elements.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 | import javax.swing.*; import java.awt.*; import java.io.*; import java.awt.event.*; class Main extends JFrame implements ActionListener { JComboBox help = new JComboBox(); JLabel txtlabel = new JLabel("Coded by saqib at mycplus.com"); JButton button1 = new JButton("First button"); JButton button2 = new JButton("Second button"); JButton button3 = new JButton("Third button"); JRadioButton radioBtn1 = new JRadioButton("Option 1"); JRadioButton radioBtn2 = new JRadioButton("Option 2"); ButtonGroup radioGroup = new ButtonGroup(); JCheckBox checkBox = new JCheckBox("Enable Feature"); //text area JTextArea output = new JTextArea("Output will show here in this GUI box", 14, 45); public Main() { super("Java Swing GUI Example"); //create window setSize(550, 500); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setVisible(true); //create content container Container contentArea = getContentPane(); contentArea.setBackground(Color.gray); //create layout manager FlowLayout flowManager = new FlowLayout(); contentArea.setLayout(flowManager); JComboBox help = new JComboBox(); help.setForeground(Color.black); help.setBackground(Color.lightGray); setContentPane(contentArea); //combo box help help.addItem("Help - Dropdown Menu"); help.addItem("-----------------"); help.addItem("1. Menu item One"); help.addItem("2. Menu item Two"); help.addItem("3. Menu item Three"); help.addItem("4. Menu item Four"); contentArea.add(help); //text label txtlabel.setBackground(Color.darkGray); contentArea.add(txtlabel); setContentPane(contentArea); output.setForeground(Color.green); output.setBackground(Color.black); contentArea.add(output); setContentPane(contentArea); //scroll pane JScrollPane scroller = new JScrollPane(output, JScrollPane.VERTICAL_SCROLLBAR_ALWAYS, JScrollPane.HORIZONTAL_SCROLLBAR_ALWAYS); scroller.setForeground(Color.darkGray); contentArea.add(scroller); setContentPane(contentArea); //buttons button1.addActionListener(this); button1.setBackground(Color.lightGray); button1.setForeground(Color.black); contentArea.add(button1); setContentPane(contentArea); button2.addActionListener(this); button2.setBackground(Color.lightGray); button2.setForeground(Color.black); contentArea.add(button2); setContentPane(contentArea); button3.addActionListener(this); button3.setBackground(Color.lightGray); button3.setForeground(Color.black); contentArea.add(button3); setContentPane(contentArea); //text label txtlabel2.setBackground(Color.darkGray); contentArea.add(txtlabel2); setContentPane(contentArea); radioGroup.add(radioBtn1); radioGroup.add(radioBtn2); contentArea.add(radioBtn1); setContentPane(contentArea); contentArea.add(radioBtn2); setContentPane(contentArea); contentArea.add(checkBox); setContentPane(contentArea); createMenuBar(); } public void actionPerformed (ActionEvent event) { if(event.getSource() == button1) output.setText("You pressed button1"); if(event.getSource() == button2) output.setText("You pressed button2"); if(event.getSource() == button3) output.setText("You pressed button3"); } private void createMenuBar() { JMenuBar menuBar = new JMenuBar(); JMenu fileMenu = new JMenu("File"); JMenuItem exitMenuItem = new JMenuItem("Exit"); exitMenuItem.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { System.exit(0); } }); fileMenu.add(exitMenuItem); menuBar.add(fileMenu); setJMenuBar(menuBar); } public static void main (String [] args) { Main eg = new Main(); } } |
Screenshot of the Swing GUI Example
When you compiler and run the above code, you will see a similar screens of the swing gui example as below.
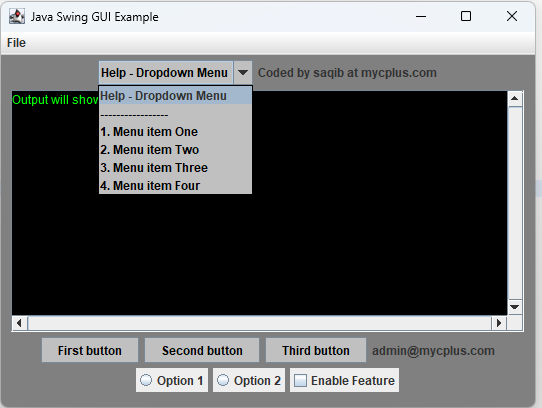
Java Swing GUI Example