This is a simple C++ program to demonstrate rock, paper, scissors game. In this game, players have to choose one of the three options to beat the opponent or the computer itself.
The following C++ code prints a simple menu showing three options to the user and then user can enter one of the options.
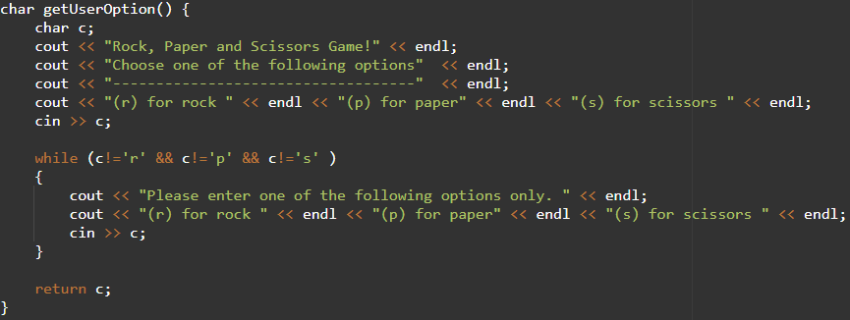
Below is the game menu which shows list of options to choose.
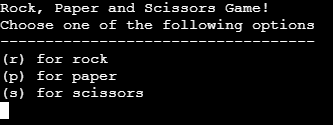
The C++ code generates a random choice by creating a character to indicate that option. Options are: r is for ‘rock’, p is for ‘paper’, and s is for ‘scissors’.
Once we have both the choices i.e. user’s choice and computer’s choice then our program compares both the choices to see who the winner is.
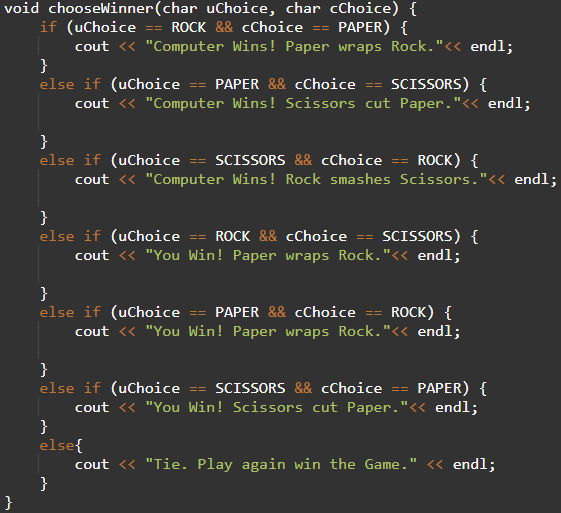
The C++ function, that compares both the choices, works based on the following criteria.
- Rock beats scissors as it smashes Scissors.
- Scissors beats paper as it cuts Papers
- Paper beats rocks as it wraps the Rock.
- Show a tie if both choices match.
Rock, Paper, Scissors Game C++ Source Code
Here is the full C++ source code for this game. Feel free to copy and paste this code into your course work/assignments and modify as necessary. In this code, computer’s choice is selected based on random number generator. We also use modulus operator and time seed to generate a different random numbers every time.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 | #include <iostream> // Constant variables const char ROCK = 'r'; const char PAPER = 'p'; const char SCISSORS = 's'; using namespace std; char getComputerOption() { srand(time(0)); // Random number int num = rand() % 3 + 1; if(num==1) return 'r'; if(num==2) return 'p'; if(num==3) return 's'; } char getUserOption() { char c; cout << "Rock, Paper and Scissors Game!" << endl; cout << "Choose one of the following options" << endl; cout << "-----------------------------------" << endl; cout << "(r) for rock " << endl << "(p) for paper" << endl << "(s) for scissors " << endl; cin >> c; while (c!='r' && c!='p' && c!='s' ) { cout << "Please enter one of the following options only. " << endl; cout << "(r) for rock " << endl << "(p) for paper" << endl << "(s) for scissors " << endl; cin >> c; } return c; } void showSelectedOption(char option) { if (option == 'r') cout << "Rock" << endl; if (option == 'p') cout << "Paper" << endl; if (option == 's') cout << "Scissors" << endl; } void chooseWinner(char uChoice, char cChoice) { if (uChoice == ROCK && cChoice == PAPER) { cout << "Computer Wins! Paper wraps Rock."<< endl; } else if (uChoice == PAPER && cChoice == SCISSORS) { cout << "Computer Wins! Scissors cut Paper."<< endl; } else if (uChoice == SCISSORS && cChoice == ROCK) { cout << "Computer Wins! Rock smashes Scissors."<< endl; } else if (uChoice == ROCK && cChoice == SCISSORS) { cout << "You Win! Paper wraps Rock."<< endl; } else if (uChoice == PAPER && cChoice == ROCK) { cout << "You Win! Paper wraps Rock."<< endl; } else if (uChoice == SCISSORS && cChoice == PAPER) { cout << "You Win! Scissors cut Paper."<< endl; } else{ cout << "Tie. Play again win the Game." << endl; } } int main() { //User's choice char uChoice; //Compter's choice char cChoice; uChoice = getUserOption(); cout << "Your choice is: "<< endl; showSelectedOption(uChoice); cout << "Computer's choice is: "<< endl; cChoice = getComputerOption(); showSelectedOption(cChoice); chooseWinner(uChoice, cChoice); return 0; } |
1
Facts about Rock, Paper and Scissors Game
1 |
The earliest known version of the game (shoushiling) might have roots in China, dating back to the Han Dynasty (approximately 206 BCE to 220 CE).
In Japan, the game is known as “jan-ken,” and it became popular during the Edo period (17th to 19th centuries).
The game gained international popularity in the 20th century, spreading to Western countries.