The following Java code defines a simple GUI application using Swing. It creates a form with text fields for name and email, a text area for messages, and actions buttons for OK and Cancel operations. Additionally, there is a button to change the background color of the form.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 | import java.awt.*; import java.awt.event.*; import javax.swing.*; public class Form extends JFrame { private JPanel bottom, top, centre; private JButton b1, b2, OK; private JLabel n, e, m; private Color color = Color.black; private String s1, s2, s3, s4 = "", s5; TextField screen = new TextField(20); TextField scr = new TextField(20); TextArea a = new TextArea(6, 20); public Form() { super("Form"); Container c = new Container(); c = getContentPane(); bottom = new JPanel(); bottom.setBorder(BorderFactory.createEtchedBorder(Color.cyan, Color.blue)); JButton b1 = new JButton("OK"); JButton b2 = new JButton("Cancel"); bottom.add(b1); bottom.add(b2); c.add(bottom, BorderLayout.SOUTH); top = new JPanel(); n = new JLabel("NAME "); e = new JLabel("EMAIL "); m = new JLabel("MESS"); top.setLayout(new FlowLayout(FlowLayout.LEFT, 200, 50)); top.add(n); top.add(scr); top.add(e); top.add(screen); top.add(m); top.add(a); c.add(top, BorderLayout.CENTER); top.setBorder(BorderFactory.createEtchedBorder(Color.blue, Color.green)); centre = new JPanel(); c.add(centre, BorderLayout.NORTH); centre.setBorder(BorderFactory.createEtchedBorder(Color.magenta, Color.pink)); Button b3 = new Button("CHANGE COL"); centre.add(b3); b3.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { Container c = getContentPane(); color = JColorChooser.showDialog(Form.this, "Choose a color", color); if (color == null) color = Color.black; centre.setBackground(color); centre.repaint(); } }); b1.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { s1 = "The name is: " + " " + scr.getText(); s1 += "\nEmail Addres is: " + " " + screen.getText(); s1 += "\nMessage is: " + a.getText(); s1 += "\nBackground Color is: " + color.toString(); JOptionPane.showMessageDialog(null, s1); } }); b2.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { dispose(); System.exit(0); } }); setSize(800, 500); setVisible(true); } public static void main(String args[]) { Form app = new Form(); app.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent e) { System.exit(0); } }); } } |
Once you run the code you will see the following Java Form.
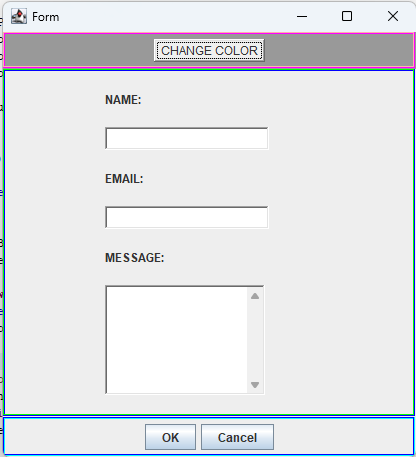
A Simple Java Form