In C Programming, operators are symbols or keywords used to perform operations on values and variables. These are fundamental to performing various operations in C programming and are essential for building complex algorithms and programs. We can use operators to perform a wide range of tasks, including arithmetic calculations, logical operations, and comparisons.
Table of Contents
- Unary Operators
- Binary Operators
- Assignment Operators ( =, +=, -=, *=, /=, %= )
- Other Assignment Operators (>>=, <<=, &=, |= and ^=)
- Arithmetic Operators (+, -, /, * and %)
- Relational or Comparison Operators (==, !=, >, <, >= and <=)
- Using both assignment (=) and comparison (==) operators together
- Logical Operators ( !, && and ||)
- Bit-wise Operators (~, &, |, ^, >> and <<)
- Ternary Operators
- Infographics – Operators Diagram
There are three major groups of operators in C which are Unary Operators, Binary Operators and Ternary Operators.
Unary Operators
In C programming, a unary operator is an operator that operates on only one operand, either to its left or right. These operators perform various operations such as incrementing or decrementing a value, negating a value, or obtaining the address of a variable.
Increment or Decrement Operators (++ and –)
The unary ++ and — operators increment or decrements the value in a variable. There are “pre” and “post” variants for both operators which do slightly different things (explained below)
1 2 3 4 5 6 7 8 9 | var++ increment "post" variant ++var increment "pre" variant var-- decrement "post" variant --var decrement "pre" variant int i = 42; i++; // increment on i // i is now 43 i--; // decrement on i // i is now 42 |
Pointer Operators (*, & and ->)
In C, pointer operators are operators that are used in conjunction with pointers. There are several pointer operators in C, including:
- Address-of operator (
&
): Returns the memory address of a variable. When used with a variable, the&
operator returns the address of the variable in memory. - Dereference operator (
*
): Accesses the value stored at a memory address. When used with a pointer, the*
operator returns the value stored at the address pointed to by the pointer. - Indirection operator (
->
): Accesses a member of a structure through a pointer. The->
operator is used to access members of a structure when a pointer to the structure is used.
Logical NOT Operator (!)
The logical NOT operator (!) is a unary operator that is used to reverse the logical state of its operand. If the operand is true, the NOT operator will make it false, and if the operand is false, the NOT operator will make it true. It essentially negates the truth value of a given expression.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | #include <stdio.h> int main() { int x = 5; // Using the logical NOT operator if (!(x == 10)) { printf("x is not equal to 10\n"); } else { printf("x is equal to 10\n"); } return 0; } |
Binary Operators
Binary operators are operators that operate on two operands. They perform operations on two values, and the result is a single value. Binary operators are used in various mathematical and logical operations. Here are some common binary operators in C:
Assignment Operators ( =, +=, -=, *=, /=, %= )
The assignment operator is the single equals sign (=).
1 2 | i = 6; i = i + 1; |
The assignment operator copies the value from its right hand side to the variable on its left hand side. The assignment also acts as an expression which returns the newly assigned value. Some programmers will use that feature to write things like the following.
1 | y = (x = 2 * x); // double x, and also put x's new value in y |
Other Assignment Operators (>>=, <<=, &=, |= and ^=)
In addition to the plain = operator, C includes many shorthand operators which represents variations on the basic =. For example “+=” adds the right hand side to the left hand side. x = x + 10; can be reduced to x += 10;. This is most useful if x is a long expression such as the following, and in some cases it may run a little faster.
1 | person -> relatives.mom.numChildren += 2; // increase children by 2 |
Here’s the list of assignment shorthand operators…
1 2 3 4 5 6 | +=, -= Increment or decrement by RHS *=, /= Multiply or divide by RHS %= Mod by RHS >>= Bitwise right shift by RHS (divide by power of 2) <<= Bitwise left shift RHS (multiply by power of 2) &=, |=, ^= Bitwise and, or, xor by RHS |
Arithmetic Operators (+, -, /, * and %)
C includes the usual binary and unary arithmetic operators. These are used to perform basic arithmetic operations such as addition (+), subtraction (-), multiplication (*), division (/), and remainder after division (%) modulo division.
Personally, I just use parenthesis liberally to avoid any bugs due to a misunderstanding of precedence. The operators are sensitive to the type of the operands. So division (/) with two integer arguments will do integer division. If either argument is a float, it does floating point division. So (6/4) evaluates to 1 while (6/4.0) evaluates to 1.5 — the 6 is promoted to 6.0 before the division.
1 2 3 4 5 | + Addition - Subtraction / Division * Multiplication % Remainder after division( modulo division) |
Relational or Comparison Operators (==, !=, >, <, >= and <=)
These operate on integer or floating point values and return a 0 or 1 boolean value to show the comparisons between two operands.
1 2 3 4 5 6 7 8 | == Equal != Not Equal > Greater Than < Less Than >= Greater or Equal <= Less or Equal To see if x equals three, write something like: if (x == 3) ... |
Using both assignment (=) and comparison (==) operators together
An absolutely classic pitfall is to write assignment (=) when you mean comparison (==). This would not be such a problem, except the incorrect assignment version compiles fine because the compiler assumes you mean to use the value returned by the assignment. This is rarely what you want
1 | if (x = 3) ... |
This does not test if x is 3. This sets x to the value 3, and then returns the 3 to the if for testing. 3 is not 0, so it counts as “true” every time. This is probably the single most common error made by beginning C programmers.
The problem is that the compiler is no help — it thinks both forms are fine, so the only defense is extreme vigilance when coding.
Logical Operators ( !, && and ||)
In Logical operators in C, the value 0 is false, anything else is true. The operators evaluate left to right and stop as soon as the truth or falsity of the expression can be deduced. (Such operators are called “short circuiting”) In ANSI C, these are furthermore guaranteed to use 1 to represent true, and not just some random non-zero bit pattern.
1 2 3 | ! Boolean not (unary) && Boolean and || Boolean or |
Bit-wise Operators (~, &, |, ^, >> and <<)
C includes operators to manipulate memory at the bit level. This is useful for writing low level hardware or operating system code where the ordinary abstractions of numbers, characters, pointers, etc… are insufficient — an increasingly rare need. Bit manipulation code tends to be less “portable”. Code is “portable” if with no programmer intervention it compiles and runs correctly on different types of computers. The bit-wise operations are typically used with unsigned types. In particular, the shift operations are guaranteed to shift 0 bits into the newly vacated positions when used on unsigned values.
1 2 3 4 5 6 | ~ Bitwise Negation (unary) – flip 0 to 1 and 1 to 0 throughout & Bitwise And | Bitwise Or ^ Bitwise Exclusive Or >> Right Shift by right hand side (RHS) (divide by power of 2) << Left Shift by RHS (multiply by power of 2) |
Do not confuse the Bit-wise operators with the logical operators. The bit-wise connectives are one character wide (&, |) while the Boolean connectives are two characters wide (&&, ||). The bit-wise operators have higher precedence than the Boolean operators. The compiler will never help you out with a type error if you use & when you meant &&. As far as the type checker is concerned, they are identical– they both take and produce integers since there is no distinct Boolean type.
Similarly the bitwise AND operator (&
) and the address-of operator (&
) serve different purposes in C.
Bitwise AND Operator (&
) is used for performing bitwise AND operation on individual bits of integers.
1 2 3 | int a = 5; // binary: 0101 int b = 3; // binary: 0011 int result = a & b; // binary: 0001 (1 in decimal) |
Address-of Operator (&
) is used to obtain the memory address of a variable.
1 2 | int x = 42; int *ptr = &x; // ptr now holds the memory address of variable x |
Ternary Operators
In C, the ternary operator, often referred to as the conditional operator, is a shorthand way of expressing an if-else statement. It is the only ternary operator in C and has the following syntax:
Conditional Operator (? :)
The conditional operator (also known as the ternary operator ) is a shorthand way of writing an if
statement. It allows you to conditionally execute an expression based on the value of a condition. The syntax of the ternary operator is as follows:
1 | condition ? expression1 : expression2 |
Comma Operator ( , )
The comma operator ,
in C is used to separate expressions. It evaluates each expression from left to right and returns the value of the rightmost expression. It is often used in places where multiple expressions are syntactically allowed but only one is expected.
For example in the following example, the expressions a++
and b++
are evaluated first and then the expression a + b
is evaluated, and the result is assigned to the variable c
.
1 2 3 4 5 6 7 8 9 10 11 12 | #include <stdio.h> int main() { int a = 5, b = 10, c; // The comma operator is used here to combine multiple expressions c = (a++, b++, a + b); printf("a = %d, b = %d, c = %d\n", a, b, c); return 0; } |
Infographics – Operators Diagram
The following diagram shows a list of operators used in C Programming.
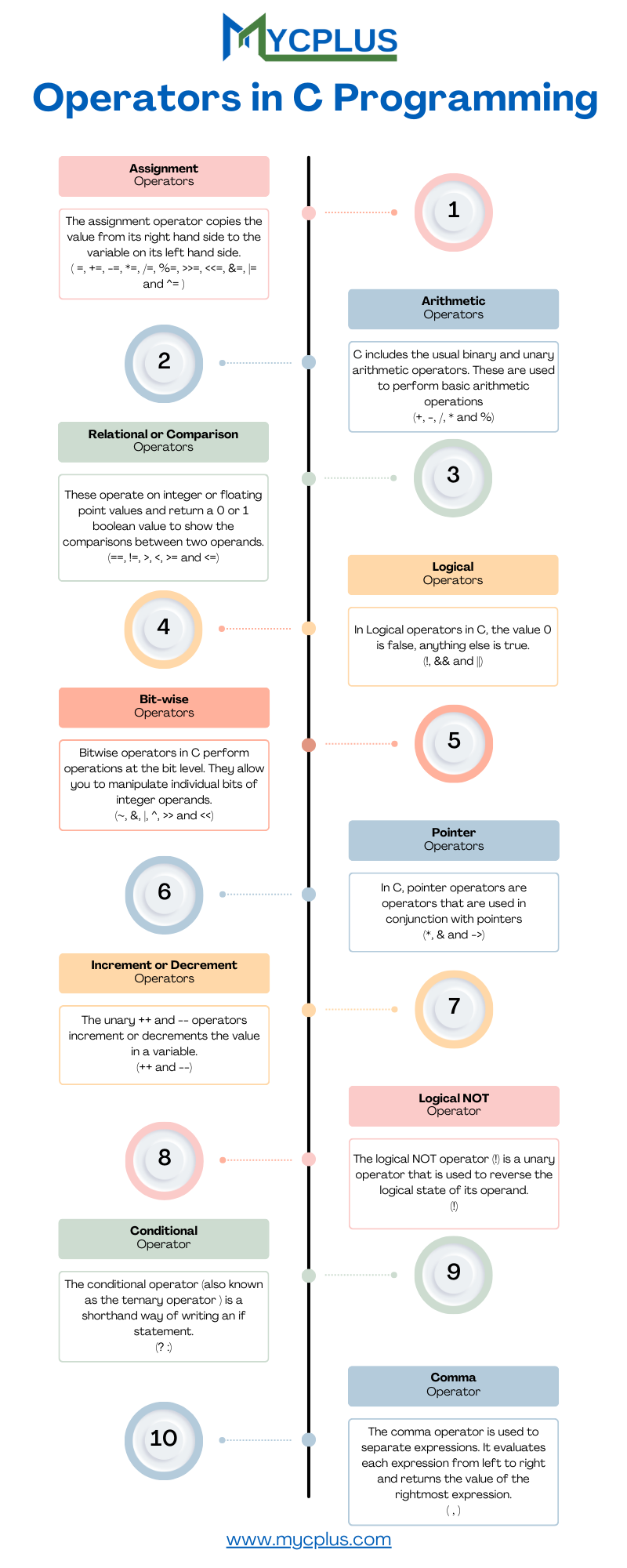
If you want to see these operators in action, look at the following C Programs: