- Introduction to Modulus Operator
- C++ Modulus Operator – C++ Modulo
- std::modulus – Specialization of Modulo
- What are the uses of Modulus Operator?
- How you can use Modulus Operator in C++?
- List Operators in C++
Introduction to Modulus Operator
The modulus operator (mod or % operator) returns the remainder of a division of one number by another. When we divide two integer numbers we will have an equation that looks like the following:
A/B = Q Remainder R where
A is the dividend
B is the divisor
Q is the quotient
R is the remainder
Sometimes, we are only interested in what the remainder is when we divide A by B. For these cases there is an operator called modulo operator (abbreviated as mod).
Using the same A, B, Q, and R as above, we would have: A mod B = R.
We would say this as A modulo B is equal to R where B is referred to as the modulus.
There are multiple uses of modulus operator such as telling if a number is a factor of another number or not. It can also be used to generate a random number or finding an even or odd number as well.
The modulus operator % can only be used with integer numbers and always have integer answer.
Below is the graphics showing different sets of numbers.
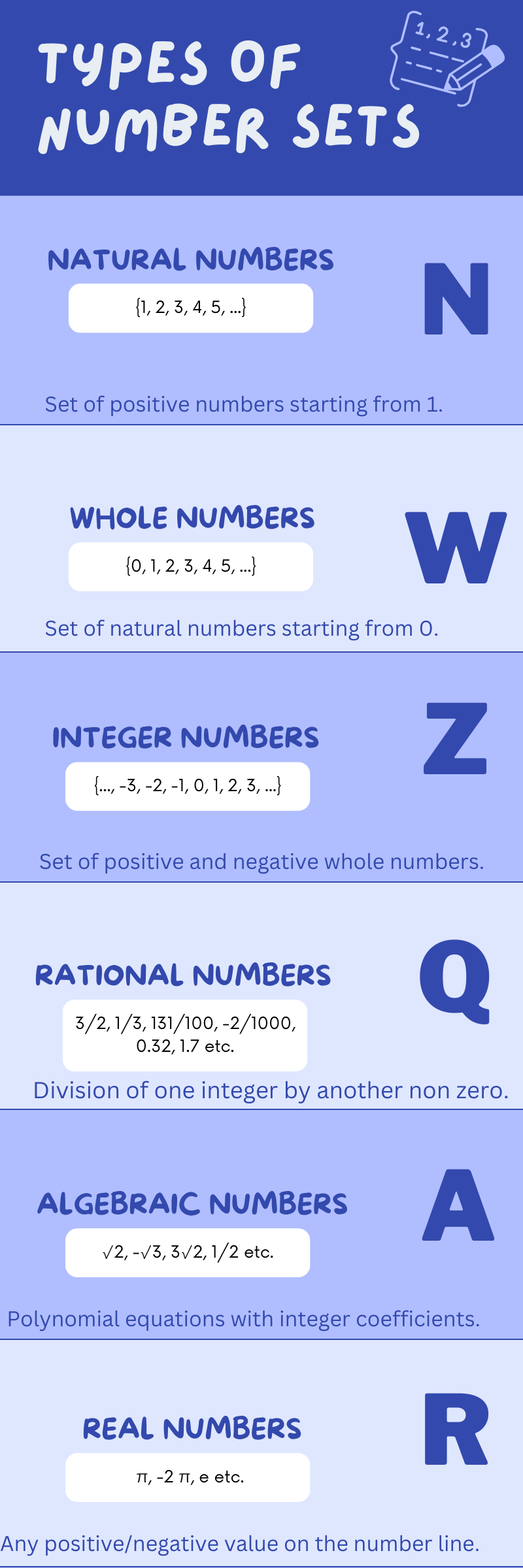
C++ Modulus Operator – C++ Modulo
How would you compute a Modulo in a programming language such as C or C++? The C/C++ provides a built-in mechanism, the modulus operator ‘%’ (percentage sign), that computes the remainder of dividing the first operand by the second. For example, 8 % 3
would return 2.
Consider the following program which takes a number from user and calculates the remainder of the number with divided by 3.
1 2 3 4 5 6 7 8 9 | #include <iostream> using namespace std; int main() { int num; cin >> num; return num % 3; } |
Take another example below which calculates whether the input number is leap year and returns 1 otherwise return 0.
1 2 3 4 5 6 7 8 9 10 11 | #include <iostream> using namespace std; int main() { int nCentury; cin >> nCentury; if ((nCentury % 4) == 0) { return 1; } return 0; } |
You may also find if a number or odd or even by using modulus operator. You can do this by asking for the remainder of the number when divided by 2.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | #include <iostream> using namespace std; int main() { int evenNum; cin >> evenNum; if (evenNum % 2 == 0 ) { cout << evenNum << " is even "; } else { cout << evenNum << " is NOT even "; } return 0; } |
You can also watch detailed video on C++ Modulus Operator below.
std::modulus – Specialization of Modulo
The C++ standard library provides a specialization of std::modulus when T is not specified, which leaves the parameter types and return type to be deduced. Binary function object class whose call returns the result of the modulus operation between its two arguments (as returned by operator %). For example, the following C++ code find whether the number in an array is even or odd by using the modulus.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | // std::cout #include <iostream> // std::modulus, std::bind2nd #include <functional> // std::transform #include <algorithm> int main () { int numbers[]={1,2,3,4,5}; int remainders[5]; std::transform (numbers, numbers+5, remainders, std::bind2nd(std::modulus<int>(),2)); for (int i=0; i<5; i++) std::cout << numbers[i] << " is " << (remainders[i]==0?"even":"odd") << '\n'; return 0; } |
1 2 3 4 5 6 7 | Output: 1 is odd 2 is even 3 is odd 4 is even 5 is odd |
What are the uses of Modulus Operator?
Knowing the remainder of a division (modulus operator) is immensely useful in lots of real life situations. For example:
- You can use modulus operator to wrap a number within a certain range/limit. For example, if you want to wrap the value of
x
within the range 0 to 10, you can usex % 10
. - Use modulus operator to determine if a number is even or odd. If
x % 2
is equal to 0, thenx
is even, otherwisex
is odd. - You can use it to repeat a loop operation a certain numbers of times by checking if the loop counter is divisible by a specific number.
- You can use modulus operator to compute the digital root of a number i.e. the sum of the individual digits of a number.
An interesting fact about modulus operator is that it is associative and distributive, meaning that you can change the order of the operands and distribute it to other operands without changing the result. For example,
(a + b) % c
is equal to(a % c + b % c) % c
.
How you can use Modulus Operator in C++?
You can use modulus operator or modulo operator in C++ programs in numbers of ways. For example you can use it to find hours, minutes and seconds from total number of seconds. You can also use it in circular array to rotate through a limited number of items. Here are two C++ programs to demonstrate the use of Modulo in real life situations.
Example 1: Find Hours, Minutes and seconds from number of seconds using C++
This is simple situation where we are given number of seconds and we can use modulo operator to find number hours, minutes and second and format it in proper time shape.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | #include <iostream> using namespace std; int main () { int seconds; int minutes; int hours; int numSeconds; cout << "Please enter number of seconds: "; cin >> numSeconds; hours = numSeconds / 3600; minutes = (numSeconds / 60) % 60; seconds = numSeconds % 60; cout << numSeconds << " seconds is: " << hours << " Hours, "; cout << minutes << " Minutes and " << seconds << " Seconds "; return 0; } |
Example 2: Rotating Through Limited Options (Circular Array) using C++
In certain situations, we might have limited number of options to go through. For example, we have three employees and one of them has to perform night shift daily, seven days of the week. So, we can use modulo operation to walk through array of days in a repeating loop.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | #include <iostream> using namespace std; int main () { // array of days that we want to cycle through string weekdays[7] = { "Mon", "Tue", "Wed", "Thu", "Fri", "Sat", "Sun" }; // couunt nummber of days in weekdays array int dayCount = sizeof( weekdays ) / sizeof( weekdays[ 0 ] ); //total number of employees int employeeCount = 15; //starting day number int dayIndex = 0; // loop through the employees while rotating through days for(int i=0; i < employeeCount; i++ ) { // employee number mod option count dayIndex = i % dayCount; // use dayIndex to find name of the day from weekdays array string weekday = weekdays[ dayIndex ]; cout << "Employee #" << i + 1 << " is scheduled on " << weekday << ". \n"; } return 0; } |
List Operators in C++
There are a numbers of built-in operator types in C and C++ and they are classified as follows and categorized into three broad categories i.e. Unary, Binary and Ternary operators. It in an essential part of C and C++ programming to understand how to use these operators correctly.
- Unary Operators
- Arithmetic Operators
- Bit-wise Operators
- Relational Operators
- Logical Operators
- Assignment Operators
- Conditional Operators
- Bitwise Operators
- Pointer Operators
Here’s Must-Read List of C programming books for beginners
C Programming Language, 2nd Edition
With over 600 5-star reviews on Amazon, readers agree that C Programming Language, 2nd Edition by Brian Kernighan and Dennis Ritchie is the best C Programming book for Beginners. The authors present the complete guide to ANSI standard C language programming. Written by the developers of C, this new version helps readers keep up with the finalized ANSI standard for C while showing how to take advantage of C’s rich set of operators, economy of expression, improved control flow, and data structures.
21st Century C: C Tips from the New School
Throw out your old ideas about C and get to know a programming language that’s substantially outgrown its origins. With this revised edition of 21st Century C, you’ll discover up-to-date techniques missing from other C tutorials, whether you’re new to the language or just getting reacquainted.
C Programming Absolute Beginner’s Guide
Greg Perry is the author of over 75 computer books and known for bringing programming topics down to the beginner’s level. His book C Programming Absolute Beginner’s Guide, is today’s best beginner’s guide to writing C programs–and to learning skills to use with practically any language. Its simple, practical instructions will help you start creating useful, reliable C code, from games to mobile apps. Plus, it’s fully updated for the new C11 standard and today’s free, open source tools!
C Programming: A Modern Approach, 2nd Edition
KN King tackles on some C standard library specifics header by header in his C Programming: A Modern Approach book. The second edition maintains all the book’s popular features and brings it up to date with coverage of the C99 standard. The new edition also adds a significant number of exercises and longer programming projects, and includes extensive revisions and updates.
Programming Arduino: Getting Started with Sketches
Simon Monk, a Ph.D. in software engineering, writes this great little book for learning to program the arduino using C language. This bestselling guide explains how to write well-crafted sketches using Arduino’s modified C language. You will learn how to configure hardware and software, develop your own sketches, work with built-in and custom Arduino libraries, and explore the Internet of Things—all with no prior programming experience required!