The book “Eloquent JavaScript, 3rd Edition” by Marijn Haverbeke is a highly regarded programming book that focuses on teaching the fundamentals of the JavaScript programming language. JavaScript is a powerful language that has revolutionized the internet by enabling dynamic and interactive web pages. The book is aimed at both beginners and experienced programmers who want to deepen their understanding of JavaScript.
Throughout the book, the author uses clear and concise explanations and includes numerous examples and exercises to help reinforce the concepts covered. The book also includes interactive exercises and quizzes on the book’s website, which provide a hands-on learning experience.
One of the unique features of the book is its approach to teaching programming concepts through projects. The author includes several projects throughout the book that progressively build on each other, providing a practical approach to learning programming.
Table of Contents
- Topics Covered in Eloquent JavaScript
- Buy this book now at Amazon and start learning JavaScript tomorrow.
- User Reviews about the book
- Our Conclusion about the Book
- Frequently Asked Questions about Eloquent JavaScript
Topics Covered in Eloquent JavaScript
The book covers a broad range of topics, from the basics of the language to more advanced topics like regular expressions and HTTP. It also includes several projects throughout the book that progressively build on each other, providing a practical approach to learning programming.
This book is divided into three parts. The first part covers the basics of the language, including data types, control structures, and functions. The second part focuses on more advanced topics, such as object-oriented programming, regular expressions, and error handling. The third part covers the browser environment, including the Document Object Model (DOM), events, and Ajax.
BUY NOW
Buy this book now at Amazon and start learning JavaScript tomorrow.
Here is the detail of each of the part covered in this book along with the topics covered in each chapter:
Part I: Language
Part I provides a solid foundation in the JavaScript programming language, covering everything from basic syntax to more advanced concepts like object-oriented programming and regular expressions. It is divided into nine chapters, each of which covers a different aspect of the language.
- Chapter 1, “Values, Types, and Operators,” introduces the basic building blocks of the language, including numbers, strings, and Booleans. It also covers operators and expressions.
- Chapter 2, “Program Structure,” covers the structure of a JavaScript program, including statements, expressions, and blocks. It also introduces control structures like if/else statements and loops.
- Chapter 3, “Functions,” covers the concept of functions in JavaScript, including function declarations and function expressions. It also covers function parameters and return values.
- Chapter 4, “Data Structures: Objects and Arrays,” covers two important data structures in JavaScript: arrays and objects. It explains how to create and manipulate arrays and objects, and how to access their properties and methods.
- Chapter 5, “Higher-order Functions,” covers functions that take other functions as arguments or return functions as values. It introduces concepts like map, reduce, and filter.
- Chapter 6, “The Secret Life of Objects,” covers the concept of object-oriented programming in JavaScript. It introduces constructors and prototypes, and how to create and inherit objects.
- Chapter 7, “Project: A Robot,” is a project that puts the concepts covered in the previous chapters into practice. It involves creating a simulation of a robot that moves around a grid.
- Chapter 8, “Bugs and Errors,” covers the common types of errors that can occur in JavaScript programs and how to debug them. It also introduces the concept of strict mode.
- Chapter 9, “Regular Expressions,” covers the concept of regular expressions and how to use them in JavaScript. It introduces the RegExp object and provides examples of how to use regular expressions to match patterns in strings.
Part II: Browser
This part provides a solid foundation in using JavaScript in the web browser environment, covering everything from manipulating the DOM to creating graphics with Canvas. The projects included in this section provide a practical approach to learning web development with JavaScript. Part II covers the basics of using JavaScript in the web browser environment. It is divided into seven chapters, each of which covers a different aspect of web development.
- Chapter 10, “JavaScript and the Browser,” provides an overview of the web browser environment and how JavaScript interacts with it. It also covers the Document Object Model (DOM), which is used to manipulate the content of web pages.
- Chapter 11, “The Document Object Model,” covers the structure of the DOM and how to use JavaScript to manipulate it. It includes examples of how to add, remove, and modify elements on a web page.
- Chapter 12, “Handling Events,” covers how to handle user events like clicks and keystrokes using JavaScript. It also covers event propagation and how to use event listeners.
- Chapter 13, “Project: A Platform Game,” is a project that puts the concepts covered in the previous chapters into practice. It involves creating a simple platform game using the DOM and event handling.
- Chapter 14, “Drawing on Canvas,” covers how to use the HTML5 Canvas element to create graphics in the web browser. It includes examples of how to draw lines, shapes, and text.
- Chapter 15, “HTTP and Forms,” covers the basics of how web forms work and how to use JavaScript to interact with them. It also covers the basics of HTTP, including HTTP methods and status codes.
- Chapter 16, “Project: A Pixel Art Editor,” is a project that puts the concepts covered in the previous chapters into practice. It involves creating a simple pixel art editor using the Canvas element and form input.
Part III: Node
Node section of the book discusses the basics of using JavaScript in the Node.js environment, which allows developers to run JavaScript code outside of the web browser. It gives solid foundation in using JavaScript using the Node.js, covering everything from module systems to asynchronous calls. The projects included in this section provide a practical approach to learning Node.js development with JavaScript. This section is divided into four chapters, each of which covers a different aspect of Node.js development.
- Chapter 17, “CommonJS,” introduces the CommonJS module system, which is used in Node.js to organize and share code between different files. It covers how to define and use modules in Node.js.
- Chapter 18, “Project: A Public Space on the Web,” is a project that puts the concepts covered in the previous chapter into practice. It involves creating a simple web server using Node.js and serving static files over HTTP.
- Chapter 19, “Asynchronous Programming,” covers the concept of asynchronous programming in Node.js, which allows developers to write code that can handle multiple tasks at once without blocking the main thread. It covers callback functions, Promises, and async/await.
- Chapter 20, “Project: Skill-Sharing Website,” is a project that puts the concepts covered in the previous chapter into practice. It involves creating a simple skill-sharing website using Node.js and a database.
User Reviews about the book
The book “Eloquent JavaScript” by Marijn Haverbeke is highly praised by many programmers for its clear and concise explanations, practical examples, and creative writing style. Many users appreciate the book’s organization and how it gradually introduces more complex topics. The projects included in the book are also well-received as they provide readers with an opportunity to apply the concepts they learned in a practical way.
Some users note that the book may not be suitable for absolute beginners, as it assumes some prior knowledge of programming concepts. Additionally, some users mention that the book focuses more on JavaScript syntax and usage than on broader web development topics like CSS and HTML.
“I have been a software developer for over 30 years, and I have read countless books on programming languages. Eloquent JavaScript is one of the best programming books I have ever read.
Amazon User
The author has a talent for explaining complex concepts in a clear and concise manner. He uses practical examples and exercises to reinforce the concepts covered, and he builds on those concepts in a logical and easy-to-follow way.
I particularly appreciated the way the author teaches programming concepts through projects. The projects are engaging and provide a practical approach to learning programming.
This book is suitable for beginners and experienced programmers alike. Even if you have been programming in JavaScript for years, I guarantee you will learn something new from this book.
I highly recommend Eloquent JavaScript to anyone looking to learn or improve their skills in JavaScript programming.”
Our Conclusion about the Book
We think that “Eloquent JavaScript, 3rd Edition” by Marijn Haverbeke is a well-written and comprehensive book that provides a solid foundation in the JavaScript language. It is an excellent resource for anyone looking to learn JavaScript or improve their understanding of the language. The book is well-organized, with clear explanations of complex concepts, practical examples, and engaging writing that keeps readers interested. The exercises and projects included in the book provide readers with an opportunity to apply what they have learned in a practical way. While the book assumes some prior programming experience, it is still accessible to beginners who are willing to put in the effort to learn. Overall, “Eloquent JavaScript, 3rd Edition” is a highly recommended resource for anyone looking to improve their JavaScript skills.
Frequently Asked Questions about Eloquent JavaScript
Is Eloquent JavaScript book suitable for beginners?
While the book does provide a thorough introduction to JavaScript, some prior programming experience is recommended. The book assumes some familiarity with programming concepts like loops, functions, and data types.
Does this book cover web development topics like HTML and CSS?
The book primarily focuses on JavaScript syntax and usage, but it does cover some web development topics like the Document Object Model (DOM), HTTP, and the HTML5 Canvas element. However, it does not cover HTML or CSS in-depth.
Are there exercises or projects included in Eloquent JavaScript, 3rd Edition?
Yes, the book includes exercises at the end of each chapter and projects at the end of each part. The exercises and projects provide readers with an opportunity to apply the concepts they learned in a practical way.
Is the book up-to-date with the latest version of JavaScript?
Yes, the book covers the latest version of JavaScript (ECMAScript 2018) at the time of its publication. However, JavaScript is a rapidly evolving language, so some of the specific details may have changed since the book’s publication.
Is there an online version of the book “Eloquent JavaScript, 3rd Edition” available?
Yes, the book is available online for free on the author’s website (eloquentjavascript.net). However, purchasing the printed version is recommended to support the author and publisher.
This book is for newbies and beginners to experienced JavaScript users.
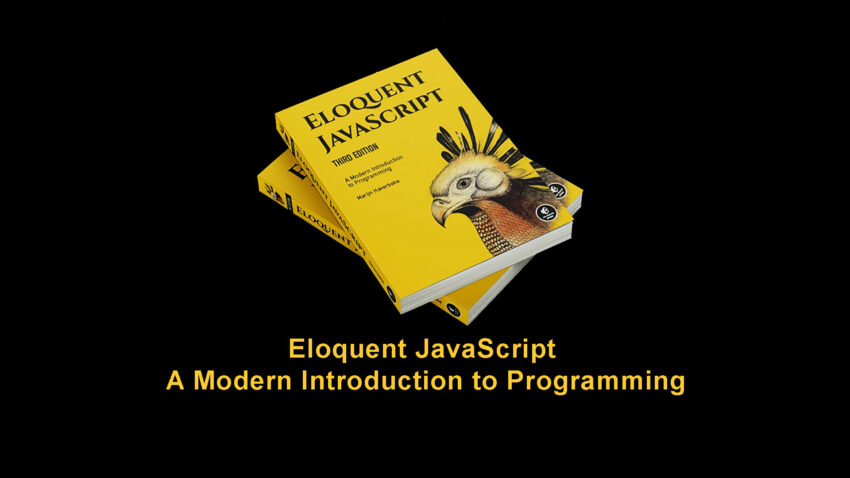