APIs, or Application Programming Interfaces, are like the building blocks of modern software and web applications. They allow different parts of a program to talk to each other. In this article, we’re going to talk about two popular types of APIs: REST and GraphQL. These are different ways of designing APIs, and choosing the right one is a big deal for any software project.
We will start by giving you the lowdown on what REST and GraphQL are, then dive into the details. We will also talk about why your choice matters so much. Your decision can affect things like how fast your software runs, how easy it is to change, and how secure it is.
So, if you are wondering which API is right for your project, keep reading and we will help you figure it out.
Overview of REST and GraphQL API Architectures
APIs come in various flavors, but two primary architectural styles have dominated the landscape: REST and GraphQL. The REST stands for Representational State Transfer which is a widely adopted approach for designing web services. It relies on a set of principles and conventions for structuring URLs and using HTTP methods for communication. Here is a REST API architecture diagram which shows different components of the REST Architecture such as client, server, resources and HTTP Methods/status codes.
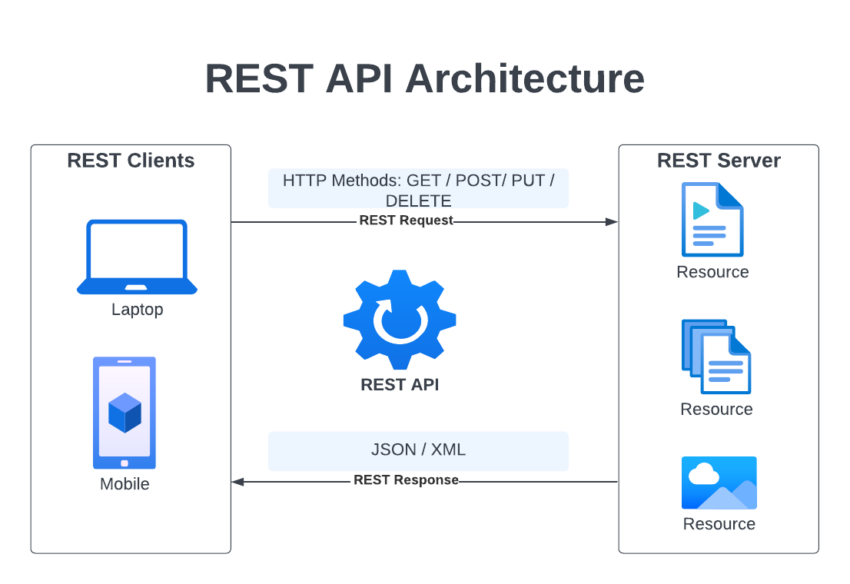
GraphQL is a more recent addition that offers a flexible way to request and manipulate data using a single endpoint. It enables declarative data fetching (based on a query) where a client can specify exactly what data it needs from an API such as an email address or name of a person. Here is a GraphQA API architecture diagram which shows different components of the API.
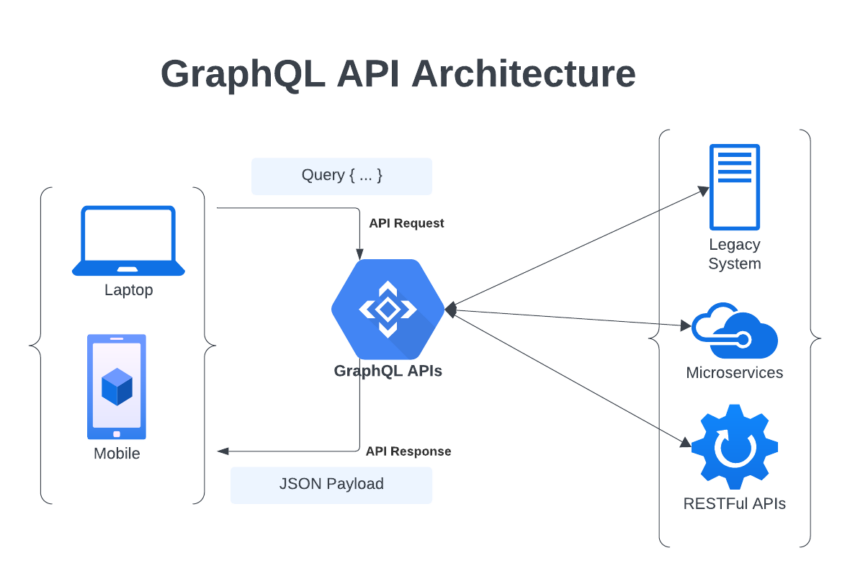
Each API Architecture has its own strengths and trade-offs, which we will discuss in this article. This will help developers and architects make informed choices when selecting the right API architecture for their projects. We compare and contrast REST and GraphQL by examining their characteristics, advantages, and disadvantages. While REST has been the go-to choice for many years, GraphQL has gained popularity for specific use cases. Our aim is to provide the insights and knowledge needed to make the best decision for your specific project requirements.
Why API Selection Matters
API selection is an important decision in software development, with far-reaching implications. The choice between REST and GraphQL affects aspects such as performance, flexibility, scalability, and ease of development. An inappropriate API choice can lead to bottlenecks, increased complexity, and future maintenance challenges. By understanding the nuances and trade-offs of these architectures, you can make decisions that align with your project’s goals and ensure its long-term success.
In the following sections, we’ll dive deeper into REST and GraphQL, analyze their strengths and weaknesses, and provide real-world examples to help you navigate the process of selecting the right API for your project.
RESTful API Architecture
REST is a widely used architectural style for designing web services. Here we discuss its principles, characteristics, how it uses HTTP methods and status codes, its resource-based routing approach, the concept of statelessness and caching, and the pros and cons associated with it.
Principles and Characteristics
REST is built on a few fundamental principles:
- Stateless Communication: Each request from a client to a server must contain all the information needed to understand and process the request. The server shouldn’t store any information about the client’s state between requests, making it easier to scale and maintain.
- Resource Based Routing: REST models the resources of an application as entities that can be identified by a unique URL. These resources can represent data objects, services, or other entities.
- Standard HTTP Methods: REST leverages standard HTTP methods such as GET (for retrieving data), POST (for creating new data), PUT (for updating data), and DELETE (for deleting data).
Statelessness and Caching
Statelessness means that each request to the server is independent and self-contained. The server does not remember previous requests. This simplicity is a strength because it reduces the chances of unexpected side effects and makes scaling easier. Additionally, REST can take advantage of HTTP caching mechanisms, allowing for faster responses when data does not change frequently.
Resource Based Routing
In a REST API, URLs are used to represent resources. The structure of these URLs is hierarchical where each part specifies a resource. For example, a URL like https://api.example.com/users/123 might be used to retrieve user number 123. This hierarchical structure makes RESTful APIs easy to understand and navigate.
HTTP Methods and Status Codes
REST APIs rely on HTTP methods to perform operations on resources. Here are some of the common HTTP methods and their purposes:
- GET: Retrieve data from the server.
- POST: Create a new resource on the server.
- PUT: Update an existing resource on the server.
- DELETE: Remove a resource from the server.
HTTP response status codes are used to indicate the outcome of an operation, such as 200 OK for a successful GET request or 404 Not Found for a resource that doesn’t exist.
Pros and Cons of REST
Some of the pros of the REST API are simplicity, scalability and compatibility.
- Simplicity: REST is easy to understand for developers and use which makes it a popular choice for many applications.
- Scalability: Stateless communication and the ability to leverage HTTP caching make it highly scalable.
- Compatibility: Works well with the existing infrastructure of the web and HTTP protocol. So, it’s a good fit for integrating with various existing web standards and http based systems and technologies
Some of the drawbacks of the REST APIs are Over/Under fetching, Versioning and Limited Flexibility.
- Over-fetching and Under-fetching: REST APIs can lead to over-fetching (retrieving more data than needed) or under-fetching (not retrieving enough data) issues.
- Versioning Challenges: Maintaining backward compatibility when making changes can be complex. As new features are added or existing ones change, it can be a challenge to ensure that old clients still work as expected.
- Limited Flexibility: In some cases, you may need to make multiple requests to retrieve related data which can lead to additional complexity in client-server interactions.
GraphQL API Architecture
GraphQL is an innovative API architecture and a query language for your API. Here we will cover the basics of GraphQL, its query language and syntax, the concept of schema definition, how resolvers work, and explore the advantages and limitations of GraphQL.
GraphQL Basics
Unlike traditional REST APIs, where the server dictates the structure of the response, in GraphQL, the client specifies exactly what data it needs. This client-centric approach makes GraphQL highly flexible and efficient.
Query Language and Syntax
GraphQL queries look like JSON objects. You request specific fields on a type, and you can also specify nested queries to fetch related data. For example, you can request user information, including their name and email, in a single query:
1 2 3 4 5 6 | { user(id: 123) { name email } } |
This query syntax allows you to get just the data you need and nothing more, reducing over-fetching and under-fetching issues.
Schema Definition
In GraphQL, you define a schema that outlines the types, queries, and mutations your API supports. The schema acts as a contract between the client and server, specifying what data can be requested and how it can be modified.
Here’s a simple example of a GraphQL schema:
1 2 3 4 5 6 7 8 | type User { id: ID name: String email: String } type Query { user(id: ID): User } |
This schema defines a User type and a user query, which can retrieve user data by their id.
Resolvers and Execution
Resolvers are functions that determine how to fetch the data requested in a GraphQL query. Each field in a query is resolved by a specific resolver. For instance, when the client asks for a user’s name, there’s a resolver responsible for fetching the name from the data source (e.g., a database). Resolvers make it possible to connect your schema to your data.
The execution of a GraphQL query involves traversing the query and calling the appropriate resolvers. The result is a JSON object that matches the shape of the query.
Pros and Cons of GraphQL
Some of the pros of the GraphQL API are efficiency, flexibility, single endpoint and strongly typed.
- Efficiency: Clients can request only the data they need so it minimizes data transfer over the network and number of requests and responses.
- Flexibility: GraphQL is highly adaptable and allows for iterative development. Unlink REST API, You can add new fields or types without breaking existing clients.
- Single Endpoint: GraphQL typically uses a single endpoint to simplify the API management.
- Strongly Typed: The schema enforces data types, making it easier to work with the API.
Some of the limitations of the GraphQL APIs are complexity, steep learning curve and caching.
- Complexity: The flexibility of GraphQL can lead to complex queries which need to be carefully managed for query execution.
- Learning Curve: Understanding GraphQL and creating efficient schemas and resolvers can be challenging.
- Caching: Caching GraphQL responses can be more complex compared to REST.
GraphQL is a powerful choice for APIs when you need flexibility, efficiency, and a client-driven data retrieval approach. It’s important to assess whether these advantages align with your project’s needs and whether you’re ready to handle the complexity that can come with it.
Comparative Analysis
In this section, we will compare REST and GraphQL to help you understand their strengths and weaknesses, and guide you in making the right choice for your project.
Performance and Efficiency
REST: REST APIs can be highly performing when used optimally, especially for read-heavy operations. However, over-fetching (retrieving more data than needed) and under-fetching (not retrieving enough data) can impact on its performance negatively.
GraphQL: GraphQL excels in performance and efficiency by allowing clients to request only the data they need. This minimizes data transfer, reducing the burden on the network and the server.
Flexibility and Versioning
REST: Making changes to a REST API can be challenging while maintaining backward compatibility. Adding or removing fields may affect existing clients, leading to versioning issues.
GraphQL: GraphQL offers outstanding flexibility. You can add, deprecate, or modify fields without breaking existing clients. This flexibility is especially valuable in agile development environments.
Data Fetching Overhead
REST: REST APIs often suffer from over-fetching, where clients receive more data than required. This can lead to wasted bandwidth and slower response times.
GraphQL: GraphQL mitigates over-fetching and under-fetching problems, as clients specify their data requirements precisely in the query. This reduces data fetching overhead.
Real-world Use Cases
REST: REST is well-suited for simple, predictable APIs and applications where data retrieval patterns are consistent. It’s a good fit for traditional web services.
GraphQL: GraphQL is designed for complex, data-intensive applications where the data requirements vary across clients. It’s particularly valuable when you have a wide range of client types and devices.
When to Choose REST?
Choose REST API architecture when:
- Your API requirements are straightforward, and you have well-defined data retrieval patterns.
- Backward compatibility is a top priority, and you want to avoid complex versioning.
- You have an existing RESTful infrastructure or when third-party integration is a primary concern.
When to Choose GraphQL?
Choose GraphQL when:
- Your application needs to optimize data transfer and reduce over-fetching and under-fetching.
- Your development team prefers an agile and flexible approach to schema changes.
- You have multiple client types with varying data needs (e.g., web, mobile, IoT).
- Complex queries, such as nested or batched requests, are common in your use cases.
Tooling and Ecosystem
Now, we will explore the tooling and ecosystem around REST and GraphQL APIs. Understanding the available frameworks, libraries, development tools, Compilers and IDEs and community support is crucial for making an informed decision.
Popular Frameworks and Libraries
REST:
- Express.js: A widely used Node.js framework for building RESTful APIs. It offers flexibility and a vast array of middleware.
- Spring Boot: A Java based framework known for its ease of use and robust REST support.
GraphQL:
- Apollo Server: A GraphQL server implementation for Node.js, known for its performance and ease of use.
- GraphQL-Java: A popular library for building GraphQL servers in Java.
Development and Testing Tools
REST:
- Postman: A popular tool for testing REST APIs which allows you to create, send, and monitor requests easily.
- Swagger: A framework for documenting and testing REST APIs, helping developers understand and interact with the API.
GraphQL:
- GraphiQL: An in-browser IDE for exploring GraphQL APIs. It provides an interactive interface for querying and testing GraphQL endpoints.
- Apollo Client Devtools: A browser extension that aids in debugging and monitoring GraphQL queries and mutations.
Community and Documentation
REST has been around for a long time, resulting in extensive documentation, tutorials, and a large community. You can find resources and solutions for a wide range of use cases.
GraphQL’s community is vibrant and growing rapidly. You’ll find ample resources, conferences, and meetups to help you learn and stay updated.
Comprehensive documentation is available from the GraphQL official website, including tutorials, best practices, and reference materials.
Future Technologies and Protocols for APIs
The world of APIs is continually evolving, and several emerging technologies and protocols are worth keeping an eye on:
gRPC: A high-performance, open-source framework for building efficient and scalable APIs. It uses HTTP/2 for transport and Protocol Buffers for serialization which makes it a strong contender for microservices architectures.
WebSockets: While not new, WebSockets are gaining traction for real-time and bidirectional communication, suitable for applications that require instant updates, like chat apps and online gaming.
WebAssembly (Wasm): Wasm is revolutionizing how code is executed on the web. It allows running code written in multiple languages at near-native speed, opening up new possibilities for API development.
Conclusion
The choice between GraphQL and REST for your project’s API is a decision that you should make carefully by taking into account the specific needs and requirements of your application. This article has provided a comprehensive comparison of both API architectures and highlighted their respective strengths and weaknesses. While REST offers simplicity, caching, and familiarity, GraphQL excels in flexibility, reduced over-fetching, and improved efficiency for complex data queries. The decision ultimately depends on factors like project complexity, data structure, and client requirements. By making an informed choice between GraphQL and REST, you can ensure that your API serves as an efficient and scalable foundation for your project.